Logging in Python
From Print Chaos to PyLogger: Revolutionizing Python Debugging
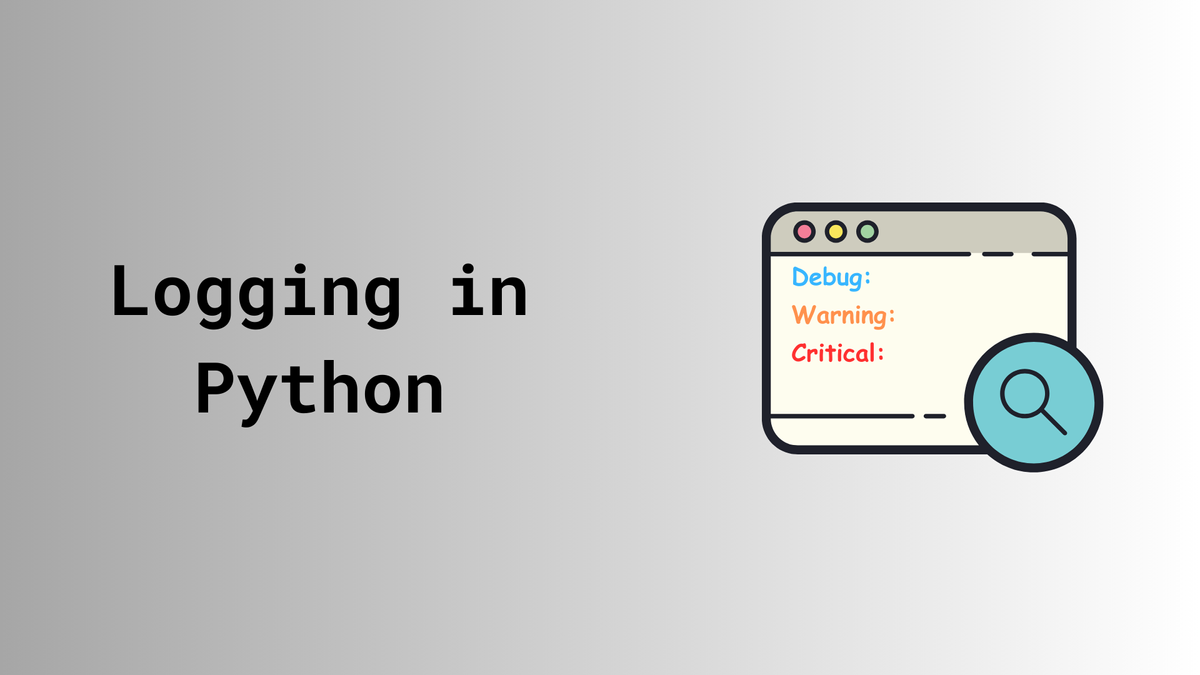
How many times have you found yourself staring at your screen, surrounded by a sea of hastily added print statements, thinking "There's got to be a better way"? If you're like me, the answer is probably "more times than I'd care to admit."
Sick of finding myself in this situation more often than I'd like, I decided to take action. Python's default logging module is powerful, but it just didn't cut it for my needs. I was looking for something more.
Why Logging Matters
Before we dive in, let's look at why logging is crucial:
- Debugging: Logs provide a trail of breadcrumbs to track down issues.
- Monitoring: They help you understand your application's behavior.
- Auditing: Logs can serve as a record of important events or actions.
- Performance Tracking: You can use logs to identify bottlenecks and optimize your code.
Introducing PyLogger
With these benefits in mind, I set out to build a custom logging class that would meet all my requirements. The result is PyLogger
, a Python logging class that aims to make logging both powerful and user-friendly.
Key Features
Below are some of the key features that make pylogger
appealing:
- Flexible Log Levels: Beyond standard levels, it includes custom levels like MESSAGE, SUCCESS, and FAILED.
- Rich Console Output: It uses the `rich` library for colorful, easy-to-read console output.
- File Logging: Capture logs to disk for long-running processes - by default logs all levels to a file.
- Environment-Aware: Respects a `CONSOLE_LOG_LEVEL` environment variable for easy verbosity adjustment to stdout.
- Detailed Context: Option to include filename and function in each log entry.
- JSON Support: Easily log complex data structures.
- Exception Handling: Log exceptions with full stack traces.
Installation
Pylogger can be installed using pip or poetry:
pip install git+https://github.com/SudarshanVK/pylogger.git@v0.1
# or
poetry add git+https://github.com/SudarshanVK/pylogger.git@v0.1
Installation
Configuration
Pylogger can be configured in several ways:
- Set the `CONSOLE_LOG_LEVEL` environment variable to control console output verbosity.
- Use the `include_caller` parameter to add function and file information to logs.
- Customize the log file path when initializing the logger.
Comparison with Standard Logging
While Python's built-in logging module is powerful, pylogger offers several advantages:
- Easier setup with sensible defaults
- Built-in rich console output
- Custom log levels for common scenarios (MESSAGE, SUCCESS, FAILED)
- Simplified JSON logging
- Easy toggling of caller information
Usage Guide
Let's walk through how to use pylogger with some practical examples:
from pylogger.pylogger import PyLogger
def main():
# Initialize the logger
logger = PyLogger("app.log")
# Basic logging
logger.debug("This is a debug message")
logger.info("This is an info message")
logger.warning("This is a warning message")
logger.error("This is an error message")
logger.critical("This is a critical message")
# Custom levels
logger.success("Operation successful")
logger.failed("Operation failed")
logger.message("Custom message")
# Logging with JSON data
data = {"name": "John", "age": 30, "city": "Melbourne"}
logger.info("User data:", data)
# Exception logging
try:
1 / 0
except Exception:
logger.exception()
# Logging with caller info
logger_with_caller = PyLogger("app.log", include_caller=True)
logger_with_caller.info("This is an info message with caller info")
main()
Sample Usage
Now lets execute the script. You will see the logs in the console and also in the file app.log
.
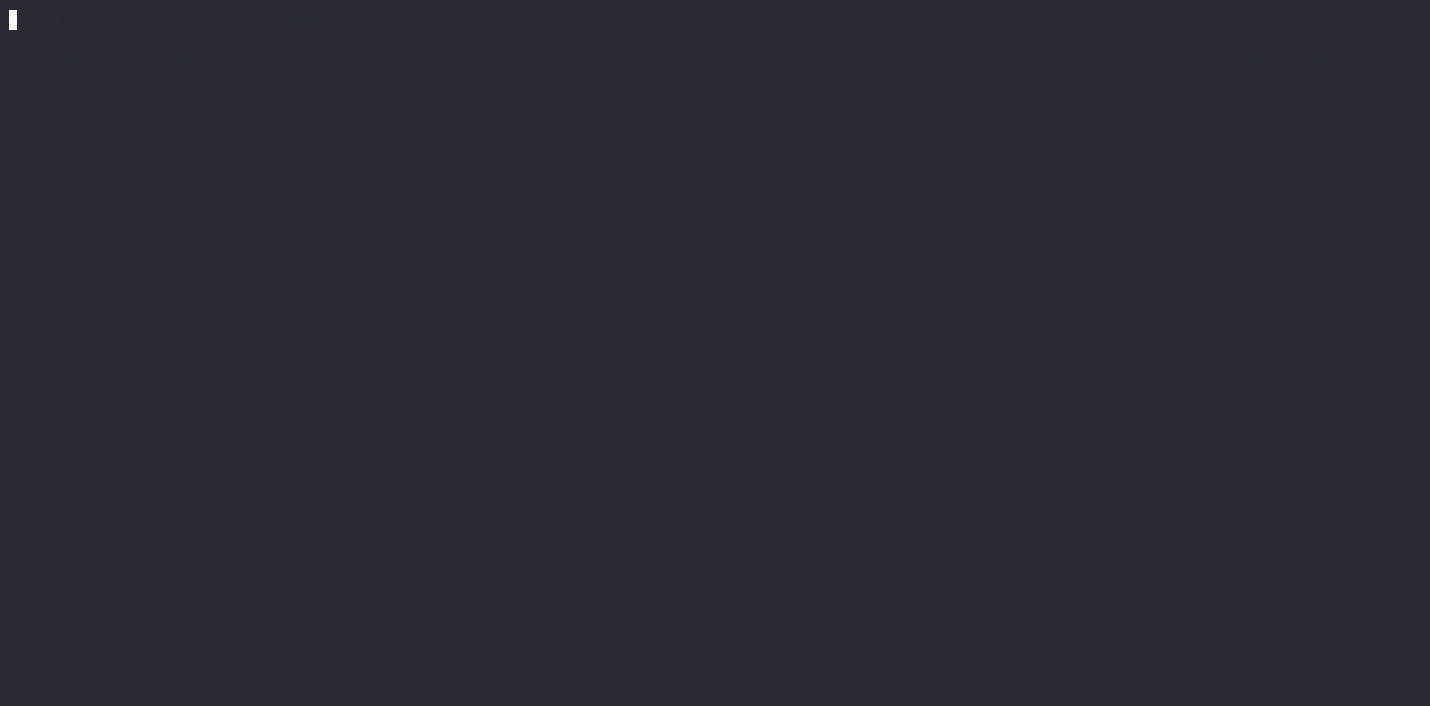
Conclusion
PyLogger
aims to make Python logging both powerful and accessible. As you use it in your projects, consider how you might extend or customize it further.
Contributions and feedback are always welcome on the GitHub repository! Happy logging, and may your debugging sessions be short and fruitful!