Network Configuration Diffing
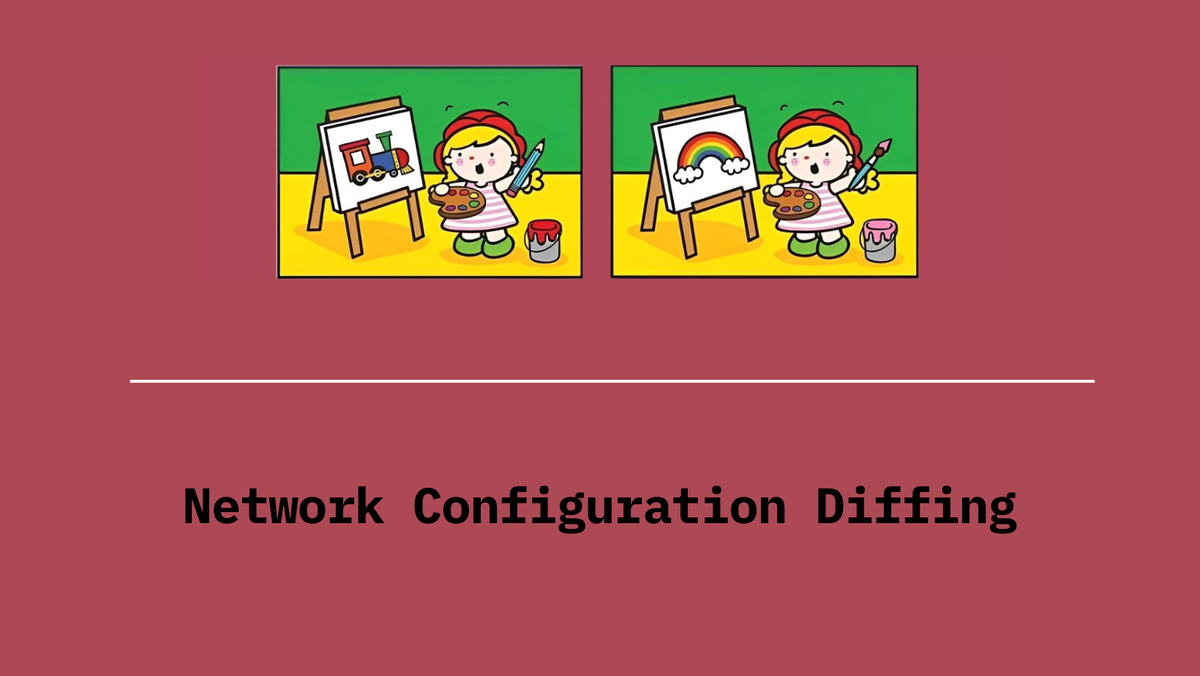
Lately, I was working on a Python script to help my team and me manage interface configurations on Cisco switches. One thing my team asked for was a way to see what changes the script was making to the configurations before and after it did its thing. My first thought was to use Python’s difflib
module for this. It’s handy for comparing two sequences and showing the differences.
To give you an idea, here’s an example of what the output looked like using the difflib
module.
import difflib
before = """
interface HundredGigE1/0/3
description Dummy interface
switchport mode access
switchport access vlan 10
end
"""
after = """
interface HundredGigE1/0/3
description Dummy interface
switchport mode access
switchport access vlan 20
end
"""
diff = difflib.ndiff(before.splitlines(), after.splitlines())
print("\n".join(diff))
The output of the above script is as below:
interface HundredGigE1/0/3
description Dummy interface
switchport mode access
- switchport access vlan 10
? ^
+ switchport access vlan 20
? ^
end
The team wasn’t too thrilled with the output because it was kinda tricky to read. You really had to know what you were looking for to make sense of it.
While looking for a suitable solution, I came across the netutils
module in python. It is important to mention that module provides a lot of other functionalities that can be used to manage network configuration and is worth exploring. Documentation to the module can be found here.
First, we install the module using pip:
pip install netutils
The netutils module provides a diff_network_config
function that can be used to compare network configurations and generate a readable diff output. The diff_network_config function takes in two network configurations and the network OS as input and returns a readable diff output.
from netutils.config.compliance import diff_network_config
before = """
interface HundredGigE1/0/3
description Dummy interface
switchport mode access
switchport access vlan 10
end
"""
after = """
interface HundredGigE1/0/3
description Dummy interface
switchport mode access
switchport access vlan 20
end
"""
network_os = "cisco_ios"
new_config = diff_network_config(after, before, network_os)
old_config = diff_network_config(before, after, network_os)
print("\n======NEW CONFIG======")
print(new_config)
print("\n======OLD CONFIG======")
print(old_config)
Executing this script we get the following output:
======NEW CONFIG======
interface HundredGigE1/0/3
switchport access vlan 20
======OLD CONFIG======
interface HundredGigE1/0/3
switchport access vlan 10
The new output is way better! It gives a clear picture of what’s going on with the configuration changes. It breaks things down nicely, showing the parent configuration (like interfaces) along with the specific interface number and the changes made to it. Definitely easier to read than the difflib module output.